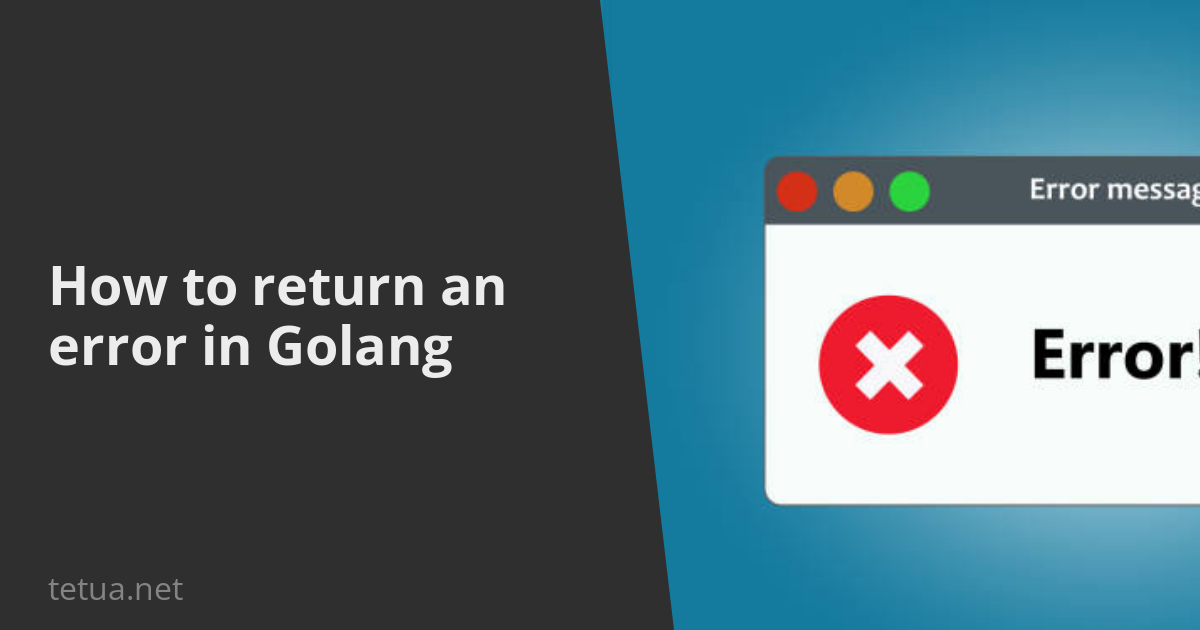
How to return an error in Golang
Introduction
Golang is a programming language created by Google. It is a statically typed language with syntax similar to that of C. It is open source and available for download.
One of the main features of Golang is that it is a compiled language, which means that it is converted into machine code that can be run on a computer. This makes Golang programs very fast and efficient.
However, Golang is not without its errors. One of the most common errors that occur in Golang is the "undefined: X" error. This error occurs when a variable or function is used that has not been defined.
There are a few ways to fix this error. The first is to simply define the variable or function that is being used. The second is to use a type assertion to convert the variable to the correct type. The "undefined: X" error can be frustrating, but it is relatively easy to fix.
What is an error in Go?
Error is a built-in type in golang. It is used to represent an error condition, and is used to indicate failure of an operation. Errors are represented by the built-in type error
. The built-in type error
is a struct type with a single field, error
, of type string
. The error
field is a string that describes the error.
An error in Go is defined as a value that implements the error interface. The error interface is a built-in interface in Go that is defined as follows:
type error interface {
Error() string
}
The error interface is used to indicate an exceptional condition that has occurred. When an error occurs, it is often useful to have some context about what caused the error. The Error()
string method provides that context.
The most common use of errors is to handle exceptional conditions when parsing text or binary data. For example, when reading a JSON document, a well-formed document must be encountered. If the document is not well-formed, an error will be returned.
Another common use of errors is to handle unexpected conditions when executing a program. For example, if a program tries to open a file that does not exist, an error will be returned.
In general, if a program encounters an error, it should be handled gracefully and not panic.
How to return an error in Go?
The Go programming language has built-in support for returning errors from functions. When a function returns an error, it is generally the last return value. The error type is a built-in interface:
type error interface {
Error() string
}
An error can be returned in any case where a regular value would be returned. When the os.Open
method fails to open a file, for example, it gives an error:
f, err := os.Open("filename.ext")
if err != nil {
// handle error
}
// use f
The err != nil
check is a common idiom in Go code. It is used to test whether the value of err is an error value or not. If it is, the code in the if block is executed.
There are many ways to create an error value. The most common is to use the New function from the errors package:
err := errors.New("something went wrong")
This creates an error with the message "something went wrong". Another common way to create an error is to use the fmt.Errorf
function:
err := fmt.Errorf("something %s", "went wrong")
This creates an error with the message "something went wrong". The %s
is a format specifier that will be replaced with the value of the "went wrong" string.
Once you have an error value, you can return it from a function like any other value:
func doSomething() error {
// ...
return fmt.Errorf("something went wrong")
}
If you want to return an error and a regular value from a function, you can use the multiple assignment idiom:
func doSomething() (string, error) {
// ...
return "", fmt.Errorf("something went wrong")
}
This idiom is often used when a function needs to return both an error and a result.
There are many functions in the Go standard library that return errors. For example, the os.Open function returns an error when it fails to open a file. When writing your own code, you should return errors wherever it makes sense to do so.